Introduction
OfficeInside 4 builds on the very basic functionality introduced in Office
Inside 3 in the form of
a "generic" class that can represent any object in the Office Object Model.
This allows the entirety of Office to be accessed.
The root of each of the Office classes is the Application, which
represents Word, Outlook, Excel or Powerpoint. Each of the OfficeInside
classes (oiWord, oiExcel, oiOutlook and oiPowerpoint) have an iApplication
member that represents this object, and allows you to interact with the
Office applications.
From there, a number of child, grandchild, and so on, classes can be accessed to
explore all the methods and properties that the various Office applications have
to offer.
Using the oiObject Class
(recommended reading)
Let's start with an example of accessing the Page Setup properties of a
Worksheet in Excel:
ExcelBook oiExcel ! Instance of the oiExcel class
iSheet oiObject
ipageSetup oiObject
code
ExcelBook.Init()
ExcelBook.iApplication.GetChild('ActiveSheet', iSheet) ! Get the active sheet from oiExcel
iSheet.GetChild('PageSetup', iPageSetup) ! Get the PageSetup for the sheet
centerFooter = 'My Worksheet' ! A string for the center footer contents
iPageSetup.Set('CenterFooter', centerFooter) ! Set the center footer to our string
This simple example demonstrates the fundamentals of the oiObject class.
Starting with the iApplication that the OfficeInside classes provide, the
GetChild method allows any of the child objects to be retrieved. These represent
the elements available in each of the Office applications. For example, in
Excel: Workbook, Worksheet, Cells, Rows etc. are all objects that can be
retrieved. In Outlook items such as Mail, Appointments, Folders and the like are
all objects that can be retrieved and interacted with. ce an object has been
retrieved, the
Get(),
Set() and
CallMethod() methods can be called.
In the example above the
Set() method is used to set a
property called '
CenterFooter' to the passed value (the
centerFooter string).
Now for a slightly more complex example, using Outlook and accessing the
Appointment item, getting and setting it's properties, anding an attendee to an
appointment.
Outlook oiOutlook
Namespace oiObject
Appointment oiObject
Recipients oiObject
Attendee oiObject
ItemID string(_oit:EntryIDSize) ! Standard Entry ID
attendeeList string(1024) ! Semi colon seperated list of attendees
code
ident = 'MAPI'
if Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
! Get the specific appointment object
if Namespace.GetChild('GetItemFromID', Appointment, ItemID)
Appointment.Set('BusyStatus', oio:Busy) ! Example of setting a property
! Get the Recipients object that is a child of the Appointment
if Appointment.GetChild('Recipients', Recipients)
! The recipients object is now fully accessible, so for example,
! Add a recipient and get the returned object so that it can be modified.
if Recipients.GetChild('Add', Attendee, attendeeName) ! Add to the recpients
Message('Attendee Added.')
Attendee.Set('Type', oio:Required)
else
Message('Failed to add an Attendee.')
end
! Other methods available for the Recpients object include:
! Add(), Item(), Remove() and RemoveAll()
Appointment.Call('Save')
! Refresh the list of attendees (C55 users should call GetProp instead of Get).
Appointment.Get('RequiredAttendees', attendeeList)
Message('Attendees: ' & Clip(attendeeList))
end
else
Message('Could not get the requested appointment, the ID may be invalid.')
end
end
! The objects can be cleaned up directly after use by calling the Kill method:
Namespace.Kill()
Appointment.Kill()
Recipients.Kill()
Attendee.Kill()
(This will be done automatically when the objects go out of scope, but for
procedures that remain open for long periods of time, this can be more
efficient.)
The oiObject class
opens up a whole host of new possibilities, by freeing you from the limitation
of what is built into the base OfficeInside classes. The oiObject class
implements the bulk of the functionality in four classes: oiWord, oiExcel,
oiOutlook and oiPowerpoint. They serve as a good basis for becoming familiar
with the Office Object Model, as well as a good place to start with your own
classes that expand on the built in classes.
A good starting place for what is available is the
Office Developer Reference.
For each of the applications (Word, Excel, Outlook and Powerpoint), see the
Object Model section under Develop Reference.
For example, for MS Word, see the
Word Object Model Reference.
Converting VBA (Macros) to Clarion
Because of the shear size of the Office Object Model it is often difficult to
determine exactly which objects are needed for a particular task. One of the
simplest approaches is to record a macro within the Office application itself. If
you're not sure how to record a macro, check the FAQ section of this doc.
For example in Word we could record a Macro for adding text and an
image to a document. Editing this Macro then displays the VBA code:
Sub quicktest()
'
' quicktest Macro
'
'
Selection.TypeText Text:="Hello World"
Selection.TypeParagraph
Selection.InlineShapes.AddPicture FileName:= _
"C:\Users\Sean\Pictures\bitshift_avatar_exp.png", LinkToFile:=False, _
SaveWithDocument:=True
End Sub
In this case the object being used is the
Selection object. The first
task is the get the
Selection object from the
Document (this isn't done in
the macro above as it is automatically provided).
All objects are a child of the Application object, and each of the
OfficeInside classes has an iApplication property which represents this
object. The GetChild method is used to get each child object. We will
start by getting the Application.ActiveDocument object and then getting
the Selection from the document:
data
Word oiWord
Document oiObject
Selection oiObject
code
Word.iApplication.GetChild('ActiveDocument', Document)
Document.GetChild('Selection', Selection)
In the code snippet above
Word is an oiWord object and both
Document
and
Selection are oiObject objects. Once the required object has been
retrieved from the parents the methods and properties can be accessed. In
the example below we have extended the oiWord class (which derives from
oiWord:
msWord.QuickTest procedure ()
Document oiObject
Selection oiObject
InlineShapes oiObject
code
!First we get a handle to the selection object that hangs off the ActiveDocument class, which is a child of the iApplication class.
if self.iApplication.GetChild('ActiveDocument', Document)
if Document.GetChild('Selection', Selection)
!In the VB macro above, we have the first line, which we will now translate into Clarion code
! Selection.TypeText Text:="Hello World"
!The method name is 'TypeText' and there is one string parameter called 'Text'
Selection.params.AddString('Hello World') !We first add the string parameter (where there are multiple params, this must be done in order)
Selection.CallEx('TypeText') !Now we call the function using CallEx
!In the VB macro above, we have the second line, which we will now translate into Clarion code
! Selection.
TypeParagraph
!The method name is '
TypeParagraph
' and in this case there are no params, so we just call the method
Selection.CallEx('TypeParagraph')
!Now we get a handle to the InlineShapes object that is a child of the Selection class
if Selection.GetChild('InlineShapes', InlineShapes)
InlineShapes.params.AddString('C:\Users\Sean\Pictures\bitshift_avatar_exp.png') !The first parameter for the AddPicture function
InlineShapes.params.AddBool(false) !The second parameter (i.e. the LinkTofile property)
InlineShapes.params.AddBool(true) !The third parameter (i.e. the
SaveWithDocument
property)
inlineShapes.CallEx('AddPicture')
end
end
end
First the Selection.TypeText method is called. Before a method is called
all the parameters required are set, in this case by calling
Selection.params.AddString(). The .params property of the oiObject class
provides parameter handling and type conversion, and allows any number of
parameters to be passed. When the parameter values are stored in variables
of the correct type the Insert method is the primary method that is used
to add parameters:
SomeFunction Function (long longVal, string stringVal, real realVal)
Selection oiObject
code
Selection.params.Insert(longVal)
Selection.params.Insert(stringVal)
Selection.params.Insert(realVal)
In addition to params.
Insert() a number of methods are
provided for explicitly type conversion, passing literal values and for
inserting "Null" values for optional parameters: AddString, AddBString,
AddInt, AddBool, AddNull.
Once the parameters have been set the CallEx method is used to call the
method and pass the parameters. The params.
Free method is
used to clear the parameters so that the next set of parameters can be
added for another method call.
Passing Parameters (oiParams)
The oiObject class provides two forms of parameter passing. The first
is for simple calls which don't take any parameters, or pass a single
integer (long), string or oiObject as a parameter. This uses the simple
Call method:
MyItem.Call('SomeMethod') ! No parameter
MyItem.Call('SomeMethod', stringVal) ! A single parameter
For more complex calls the oiObject.CallEx() method allows any number
of parameters of any types to be passed. It also supports optional
parameters, which can be passes as "Null". The oiObject.params property
allows these parameters to be specified. This property is an instance of
the oiParams class and provides methods for setting the parameters to
pass.
When the parameter values are stored in variables of the correct type
the Insert method is the primary method that is used to add parameters:
SomeFunction Function (long longVal, string stringVal, real realVal)
Selection oiObject
code
Selection.params.Insert(longVal)
Selection.params.Insert(stringVal)
Selection.params.Insert(realVal)
In addition to params.
Insert() a number of methods are
provided for explicit type conversion, passing literal values and for
inserting "Null" values for optional parameters:
AddString
AddString (string sVal, long pType = 0)
Description
Adds the passed string as a parameter. Allows the COM parameter type to
be specified by setting pType to one of the following values:
oiParam:String equate(0) ! string
oiParam:Long equate(1) ! integer (Clarion long)
oiParam:Bool equate(2) ! boolean (Clarion bool)
oiParam:Real equate(3) ! float (Clarion real)
oiParam:Short equate(4) ! Short
oiParam:Const equate(5) ! A Constant (equate).
AddBString
AddBString (string bs)
AddInt
AddInt (long pVal, bool isBool=false)
AddBool
AddBool (long pVal)
AddNull
AddNull ()
Class Methods
Methods provided by the oiObject class, along with examples demonstrating the
typical use of each method.
Construct | The object Constructor |
Destruct | The object Destructor |
Init | Intializes the object as a new object of the selected type |
Kill | Cleans up and clears the object |
IsInitialized | Checks whether the object has been initialized |
GetChild | Retrieves a child object of the current object by name |
GetChildEx | Retrieves a child object and allows parameters to be passed |
GetParent | Returns the Parent of this object |
MoveTo | Moves this object into another oiObject |
AsVariant | Returns the address of a Variant that represents the object |
FromVariant | Creates a new object from a Variant which stores an object |
Get | Retrieves a property of the object |
GetType | Retrieves the type of a property of the object |
GetTypeName | Retrieves a description of the type passed |
Set | Sets a property of the object |
SetString | Set a property and pass a string literal |
SetInt | Set a property and pass a long value |
SetBool | Set a property and pass a Office Boolean value |
Call | Call a method of the object |
CallEx | Call a method of the object |
Construct
Construct ()
Description
The class constructor, called when the object enters scope.
Parameters
None
Return Value
None
Notes
This method should not be called directly. Use the
Init
or
GetChild method to
get a specific object.
See Also
Kill,
Init,
GetChild
Destruct
Destruct ()
Description
The class destructor, handles cleanup when the object is no longer in scope.
The
Kill method can be used to deallocated memory before the object goes to of
scope. Objects can be resused by calling Kill before reusing them.
Parameters
None
Return Value
None
Notes
This method should not be called directly. Use the
Kill method to cause the object to clean up and deallocate memory.
See Also
Kill,
Init,
GetChild
ErrorTrap
ErrorTrap ()
Description
The ErrorTrap method is called when an error occurs. Additional information
is passed to the ErrorTrap method, and it provides centralised error handling.
In addition the method sends debug and error information to the system debug
output if the .debug property of the class is set to True (1) and if the
application was run using one of the OfficeInside debug command line switches
such as /oidebugmax.
Parameters
None
Return Value
None
Notes
This method is a callback method and is
not typically called directly (member of the object will call this method to
trap errors).
See Also
Kill,
Init,
GetChild
Init
Init (string pName, *oiObject iParent, <long itemNumber>), long, proc
Description
Initialises the object and associates it with the Outlook Object Model object
based on the name specified and the parent object passed. Each of the core
OfficeInside objects has an iApplication property, which represents the Office
application associated with that object. This is typically used as the parent
object for retrieving other objects in the Office Object Model heirarchy.
As an alternative to the Init method, the
GetChild method is provided.
Functionaly the two methods are identical, however
GetChild provides
alternative syntax that can improve code legibility. The
GetChild method also
provide additional functionality, allowing string and long parameters. We
recommend using GetChild rather than Init in most cases.
Parameters
Parameter |
Description |
string pName | The name of object to retrieve from the parent. |
*oiObject iParent, | The parent object to get the child from. |
<long itemNumber> | A optional long that identifies which item should be
retrieved. This is typically used when retrieving an item from a collect of
multiple objects (for instance when getting a specific document from a
collection of documents). |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Appointment oiObject
Recipients oiObject
code
Recipients.Init('Recipients', Appointment)
! Alternative using GetChild to initialise the object:
Appointment.GetChild('Recipients', Recipients)
|
See Also
GetChild
Kill
Kill ()
Description
Cleans up the object and deallocates any memory. This is called automatically
by the destructor when the object is no longer in scope. The Kill method can be
called to clean up before the object is out of scope. It can also be used to
clean up before call the Init or GetChild method to reuse the oiObject object
(see the example below).
Parameters
None
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
Example |
oItem oiObject
code
Namespace.GetChild('GetItemFromID', oItem, ItemID) ! Get a item from Outlook
! Note: The ItemID passed is an Outlook ID that uniquely identifies and item
! previously added or retrieved.
oItem.Call('Save') ! Call the Save method
Appointment.Set('BusyStatus', oio:Busy) ! Example of setting a property
oItem.Kill() ! Clean up
! The object can now be reused as needed
ExcelBook.iApplication.GetChild('ActiveSheet', oItem) ! Get the active sheet from oiExcel
oItem.Call('Delete') ! Delete the sheet
oItem.Kill() ! Clean up
|
See Also
GetChild ,
Init
GetChild
GetChild (string cName, *oiObject child, <*long itemNumber>), long, proc
GetChild (string cName, *oiObject child, *string identifier), long, proc
Description
Retrieves a child object based on the name specified. The Office Object model
is hierarchical, with the Application object at the root. This allows a child
object to be retrieved from the parent. Once an object is retrieved the methods
and properties can be accessed, along with any child objects that the new object
provides. For example the Documents object is a child of the Word Application
object. The Documents object contains a number of Document children, and each
Document contains child object that represent text , tables, selections and the
like.
Parameters
Parameter |
Description |
string cName | The name of the child object to retrieved. |
*oiObject child, | The object to store the child in. |
<*long itemNumber> | An optional parameter that specifies the position of the
child object in a collection of objects. |
*string identifier | An optional parameter that specifies the identifier of the
child object in a collection of objects. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Outlook oiOutlook
Namespace oiObject
Appointment oiObject
Recipients oiObject
code
oiOutlook.Init() ! Initialise the Outlook Object
ident = 'MAPI' ! Get the NameSpace Child
Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
! Get the specific Appointment child object
Namespace.GetChild('GetItemFromID', Appointment, ItemID)
! Get the Recipients object that is a child of the Appointment
Appointment.GetChild('Recipients', Recipients)
|
See Also
Init,
Kill,
GetParent
GetParent
GetParent (*oiObject iParent), long, proc
Description
Retrieves the parent oiObject, if there is one associated
with the current object.
Parameters
Parameter |
Description |
*oiObject iParent | An oiObject that will hold the parent object. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Appointment oiObject
Recipients oiObject
code
Recipients.GetParent(Appointment)
|
See Also
GetChild
FromVariant
FromVariant (*oiVariant vtRet), long
Description
Initializes the object using the passed variant. The
variant contains an COM object. This method is not typically called directly, in
normal use the GetChildEx method
provides the ability to retrieved
Parameters
Parameter |
Description |
vtRet | An oiVariant which contains a COM
object, such as that returned by the Call,
CallEx or Get methods |
Return Value
Returns True (1) for success or False (0)
for failure.
Examples
Example |
Appointment oiObject
varObj like(oiVariant)
code
oItems.pItems.AddInt(1)
! Option1: Get the child object as a Variant and convert it manually
if oItems.CallEx('Item', , varObject) ! Get the item from the Items collection
Appointment.FromVariant(varObject) ! Convert the returned variant to an object
end
! Option 2: Use GetChildEx to convert it internally
oItems.GetChildEx('Item', Appointment)
|
See Also
None
AsVariant
AsVariant (), long
Description
Returns a long that represents the underlying object as a variant. This is
used internally to pass objects as parameters. It is not typically used
directly.
Parameters
None
Return Value
Returns the address of a variant that contains the object if
successful, and zero if a failure occurs..
Examples
Example |
Appointment oiObject
varObj long
code
varObject = Appointment.AsVariant()
|
See Also
None
Get
Get (string propertyName, *long pValue), long, proc
Get (string propertyName, *string pValue), long, proc
Get (string propertyName, *long dateValue, *long timeValue), long, proc
Get (string propertyName, *oiVariant pValue), long, proc
Get (string propertyName, *short pValue), long, proc
Get (string propertyName, *real pValue)
Description
Gets a property from an object using the name of the property.
Note: For Clarion 5.5 users these methods are named GetProp, as the C55
compiler does not allow Get as a method name.
Parameters
Parameter |
Description |
string propertyName | The name of the property to retrieve. |
The following additional parameter options are supported: |
pValue | The variable to receive the specified value. Office
Inside handles the necessary type conversion internally. |
*long dateValue, *long timeValue | When retrieving a date/time,
the object splits the Office date/time into a Clarion date and time and
stores the values in the passed Long variables. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occuring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Appointment oiObject
NameSpace oiObject
ident string(200) ! Outlook Item Identifier
attendeeList string(4096)
code
ident = 'MAPI'
if Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
if Namespace.GetChild('GetItemFromID', Appointment, ItemID)
Appointment.Get('RequiredAttendees', attendeeList)
end
end
|
See Also
Set,
Call
GetType
GetType (string propertyName), long
Description
Returns a long that contains the TYPE for the property that matches the
passed propertyName. This can be used to identify the type of a particular
property of an object. Use the
GetTypeName method to convert
the returned integer into
Parameters
Parameter |
Description |
propertyName | The name of the property to fetch the type of. |
Return Value
Returns an integer for the type of the property, or -1 if an
error occurs.
Examples
Example |
Appointment oiObject
varObj long
code
varObject = Appointment.AsVariant()
|
See Also
None
GetType
GetType (string propertyName), long
Description
Returns a long that contains the TYPE for the property that matches the
passed propertyName. This can be used to identify the type of a particular
property of an object. Use the
GetTypeName method to convert
the returned integer into
Parameters
Parameter |
Description |
propertyName | The name of the property to fetch the type of. |
Return Value
Returns an integer for the type of the property, or -1 if an
error occurs.
The following TYPES are defined (Call GetTypeName to
convert the TYPE into a string that contains the type description).
oiVARENUM itemize(0), pre(oi)
VT_EMPTY equate(0)
VT_NULL equate(1)
VT_I2 equate(2)
VT_I4 equate(3)
VT_R4 equate(4)
VT_R8 equate(5)
VT_CY equate(6)
VT_DATE equate(7)
VT_BSTR equate(8)
VT_DISPATCH equate(9)
VT_ERROR equate(10)
VT_BOOL equate(11)
VT_VARIANT equate(12)
VT_UNKNOWN equate(13)
VT_DECIMAL equate(14)
VT_I1 equate(16)
VT_UI1 equate(17)
VT_UI2 equate(18)
VT_UI4 equate(19)
VT_I8 equate(20)
VT_UI8 equate(21)
VT_INT equate(22)
VT_UINT equate(23)
VT_VOID equate(24)
VT_HRESULT equate(25)
VT_PTR equate(26)
VT_oiSafeArray equate(27)
VT_CARRAY equate(28)
VT_USERDEFINED equate(29)
VT_LPSTR equate(30)
VT_LPWSTR equate(31)
VT_RECORD equate(36)
VT_FILETIME equate(64)
VT_BLOB equate
VT_STREAM equate
VT_STORAGE equate
VT_STREAMED_OBJECT equate
VT_STORED_OBJECT equate
VT_BLOB_OBJECT equate
VT_CF equate
VT_CLSID equate
VT_VERSIONED_STREAM equate
VT_BSTR_BLOB equate(0fffh)
VT_VECTOR equate(01000h)
VT_ARRAY equate(02000h)
VT_BYREF equate(04000h)
VT_RESERVED equate(08000h)
VT_ILLEGAL equate(0ffffh)
VT_ILLEGALMASKED equate(0fffh)
VT_TYPEMASK equate(0fffh)
end
Examples
The example below reads the type of the Zoom
property of the Excel PageSetup object. If this is a Boolean value then it
means that zooming is disabled and the Fit To Pages options are being
used. If it is an integer it means that it is enabled. This example
switches the value. Properties with the "dual nature" are fairly uncommon,
however
GetType and
GetTypeName are
still immensely useful for debugging.
Example |
propType long
code
Excel.GetPageSetup()
propType = Excel.PageSetup.GetType('Zoom')
Message('The Zoom property type is ' & Appointment.GetTypeName(propType))
case propType
of oi:VT_BOOL ! If it's currently a Bool it means it is disabled, set it to a Long value to enable it
Excel.Pagesetup.SetInt('Zoom', 96) ! Switch to using a Zoom (96%)
of oi:VT_I4
Excel.Pagesetup.SetBool('Zoom', oi:False)
Excel.Pagesetup.SetInt('FitToPagesWide', 1)
Excel.Pagesetup.SetInt('FitToPagesWide', 1)
end
|
See Also
None
GetTypeName
GetTypeName (long pType), string
Description
Returns a string that contains a description of the TYPE specified by the
pType paramter. Call the GetType method to get the TYPE for a particular
property.
Parameters
Parameter |
Description |
propertyName | The name of the property to fetch the type of. |
Return Value
Returns an integer for the type of the property, or -1 if an
error occurs.
Examples
Example |
Appointment oiObject
varObj long
code
varObject = Appointment.AsVariant()
|
See Also
None
Set
Set (string propertyName, *real pValue), long, proc
Set (string propertyName, *string pValue), long, proc
Set (string propertyName, *long pValue), long, proc
Set (string propertyName, *short pValue), long, proc
Set (string propertyName, long dateValue, long timeValue), long, proc
Set (string propertyName, long pValue), long, proc
Set (string propertyName, *oiObject pObj), long, proc
Description
Sets the value of a property of an object.
Note: For Clarion 5.5 users these methods are named
SetProp, as the C55 compiler does not allow Set as a method name.
Parameters
Parameter |
Description |
string propertyName | The name of the property to set the value for. |
The following additional parameters options are supported: |
pValue | The variable that contains the value to set the property
to, or |
dateValue, timeValue | The Clarion date and time to set the DateTime property to.
The date and time will be automatically converted to the Office DateTime format,
or |
pObj | An oiObject object that represents an object to set the
property to (this is infrequently used and handles the case where a property
being set is itself and object). |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occuring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Appointment oiObject
NameSpace oiObject
ident string(200) ! Outlook Item Identifier
code
ident = 'MAPI'
if Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
if Namespace.GetChild('GetItemFromID', Appointment, ItemID)
Appointment.Set('BusyStatus', oio:Busy) ! Example of setting a property
end
end
|
See Also
Get,
Call,
SetInt,
SetString,
SetBool
SetInt
SetInt (string propertyName, long pValue), long, proc
Description
Sets the value of a property
of an object and passes an integer by value (allowing
constants or literal values to be passed). Note that for Boolean values the
SetBool method should be called and the Office Boolean value
should be used (
oi:True and
oi:False)
not the Clarion boolean values.
Parameters
Parameter |
Description |
propertyName | The name of the property to set the value for. |
pValue | The value to set the Property to. |
Return Value
Returns True is successful or False if an error occurs.
SetBool
SetBool ((string propertyName, long oiBool), long, proc
Description
Sets the value of a property
of an object where the property is a Boolean type
(msoTristate). The Office Boolean values should be used (
oi:True and
oi:False))
not the Clarion boolean values.
Parameters
Parameter |
Description |
propertyName | The name of the property to set the value for. |
oiBool | The value to set the property to. The
Office Boolean values should be used (oi:True and oi:False))
not the Clarion boolean values. |
Return Value
Returns True is successful or False if an error occurs.
SetString
SetString (string propertyName, string pValue), long, proc
Description
Sets the value of a property
of an object and passes the string by value, allowing
contants, equates and literals to be passed.
Parameters
Parameter |
Description |
propertyName | The name of the property to set the value for. |
pValue | The value to set the Property to. |
Return Value
Returns True is successful or False if an
error occurs.
Call
Call procedure (string methodName), long, proc
Call procedure (string methodName, *long pValue), long, proc
Call procedure (string methodName, *string pValue), long, proc
Call procedure (string methodName, *oiObject pObj), long, proc
Description
Calls a method that belongs to the current object. The
oiObject must be intialised by calling Init, or by passing it to the GetChild
method of the parent, before this functionality can be called.
Parameters
Parameter |
Description |
string methodName | The name of the method to call. |
*long pValue, or *string pValue,
or *oiObject pObj | Optionally a value can be passed to the called method. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Appointment oiObject
NameSpace oiObject
ident string(200) ! Outlook Item Identifier
code
ident = 'MAPI'
if Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
if Namespace.GetChild('GetItemFromID', Appointment, ItemID)
Appointment.Call('Save') ! call the Save method
end
end
|
See Also
CallEx,
Call (multi parameter)
Call
Call (string methodName, *oiObject pObj), long, proc
Description
Calls a method that belongs to the current object. The
oiObject must be intialised by calling Init, or by passing it to the GetChild
method of the parent, before this functionality can be called.
Parameters
Parameter |
Description |
string methodName | The name of the method to call. |
*long pValue, or
*string pValue, or
*oiObject pObj | Optionally a value can be passed to the called method. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
Example |
Appointment oiObject
NameSpace oiObject
ident string(200) ! Outlook Item Identifier
code
ident = 'MAPI'
if Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
if Namespace.GetChild('GetItemFromID', Appointment, ItemID)
Appointment.Call('Save') ! call the Save method
end
end
|
See Also
CallEx,
Call (multi parameter)
Call
Call (string methodName, *oiParams pParams, long memberType = oi:DISPATCH_METHOD, <*oiVariant
vtRet>), long, proc
Description
Calls a method that belongs to the current object. The
oiObject must be intialised by calling Init, or by passing it to the GetChild
method of the parent, before this functionality can be called. This version of
the call Method allows any number of parameters to be passed using an oiParams
object. This method is not typically used - generally the CallEx method is used
to provide this functionality (the methods are identical in function, this
method simply allows an oiParams object to be passed rather than using the
oiObject.params object).
Parameters
Parameter |
Description |
string methodName | The name of the method to call. |
pParams | An oiParams object which contains all the parameters to
pass. Use the oiParams.Insert and oiParams.Add methods to add parameters to a
call. |
memberType | The type of member being called. By default this is calls a
method of the object, however multiple parameters can also be passed when
getting and setting properties (although this is unusual and seldom used). Can
be one of the following values:
oi:DISPATCH_METHOD - Call a method
oi:DISPATCH_PROPERTYGET - Get a property
oi:DISPATCH_PROPERTYPUT - Put (Set) the value of a property
oi:DISPATCH_PROPERTYPUTREF - Put a property that is a reference |
vtRet [optional] | An optional oiVariant group. If this is passed then the
value returned from the call will be stored in this group. A variant can
represent any type of data, including objects. For methods which return an
object the variant can be passed to an oiObject's FromVariant method to create a
new oiObject which represent that object contained in the variant. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
See Also
CallEx
CallEx
CallEx (string methodName, long memberType = oi:DISPATCH_METHOD, <*oiVariant vtRet>), long, proc
Description
Calls a method that belongs to the current object. This
version of the call Method allows any number of parameters to be passed using
the oiObject.params member, which can be used to add any number and type of
parameters to a call.
The oiObject must be intialised by calling Init, or
by passing it to the GetChild method of the parent, before this
functionality can be called.
Parameters
Parameter |
Description |
string methodName | The name of the method to call. |
memberType | The type of member being called. By default this is calls a
method of the object, however multiple parameters can also be passed when
getting and setting properties (although this is unusual and seldom used). Can
be one of the following values:
oi:DISPATCH_METHOD -
Call a method
oi:DISPATCH_PROPERTYGET - Get a property
oi:DISPATCH_PROPERTYPUT - Put (Set) the
value of a property
oi:DISPATCH_PROPERTYPUTREF - Put a
property that is a reference |
vtRet [optional] | An optional oiVariant group. If this is passed then the
value returned from the call will be stored in this group. A variant can
represent any type of data, including objects. For methods which return an
object the variant can be passed to an oiObject's FromVariant method to create a
new oiObject which represent that object contained in the variant. |
Return Value
Returns True (1) for success and False (0) for failure. In
the event of an error occurring the
ErrorTrap method will be
called with additional information.
Examples
The example below calls the Word.Documents.Add
method. It demonstrates getting the Documents object from the Word
application and then calling the Add method, passing four parameters, one
of which is optional. Uses an
oiWord object called Word.
When multiple calls are made using the same .params object call
FreeParams() between each call to clear the values stores.
Example |
! Create a new document. Uses the Word.Documents interface
! to add a new document in Word.
!
! Parameters
! template: The name of the template to be used for the new document.
! If this argument is omitted, the Normal template is used.
! newTemplate: oi:True to open the document as a template. The default value is oi:False.
! documentType: Can be one of the following NewDocumentType constants:
! oiw:NewBlankDocument, oiw:NewEmailMessage, oiw:NewFrameset, or oiw:dNewWebPage.
! The default constant is oiw:NewBlankDocument
! visible: oi:True to open the document in a visible window. If this value is oi:False,
! Microsoft Word opens the document but sets the Visible property of the
! document window to oi:False (hidden). The default value is oi:True.
! Return Value
! Returns True for success of False for failure.
NewDoc Procedure(<string template>, long newTemplate = oi:False, long documentType=oiw:NewBlankDocument, long visible=oi:True)
Documents oiObject
code
if not Word.iApplication.GetChild('Documents', Documents)
return False
end
! Call: Application.Documents.Add(template, newTemplate, documentType, visible)
Documents.params.Free()
if Omitted(2)
Documents.params.AddNull()
else
Documents.params.Insert(template)
end
Documents.params.AddBool(newTemplate)
Documents.params.Insert(documentType)
Documents.params.AddBool(visible)
return Documents.CallEx('Add')
|
See Also
Call
Class Properties
The properties provided by the class. Other than the name property, the class
does not currently provide any properties that can be accessed.
handle | Internal handle to the interface |
parent | Internal handle to the parent interface |
name | The name of this object such as "Cell" or "Range" |
params | oiParams object, used for calls with any number of parameters |
name | string(64)
| The name of this object such as "Cell" or "Range".
This is set by the object after the Init or GetChild method is used to associate
the oiObject with an object in the Office Object Model. It is provided primarily
for debugging and convenience. Setting it directly has no effect. |
How Tos and Examples
Also see the Generics example application, and the example code provided above
for each of the methods.
See the
CallEx
method for additional information and example code.
How To: Pass any number of parameters of any type to a method (or property Get/Set call):
! Inserts the contents of a file into the current document (merging it
! with the document).
! Parameters
! fileName: The name of the file to insert the contents of.
! Notes:
! This calls Selection.InsertFile(fileName, range, confirm, link, attachment)
! The call used in this method merges the file with the current document - i.e.
! it inserts the contents at the end of the current document. The Selection.InsertFile
! method can also be used to insert the contents of a document at a particular
! position (range), as well as it link or attach a document rather than inserting
! it directly.
msWord.InsertFile Procedure(string fileName)
code
if not self.Selection.IsInitialized()
Message('Error, there was no Selection object available. Cannot Insert the file', 'Word File Insertion Error')
return False
end
self.Selection.params.Free()
self.Selection.params.AddString(Clip(fileName))
self.Selection.params.AddNull()
self.Selection.params.AddBool(oi:False)
self.Selection.params.AddBool(oi:False)
self.Selection.params.AddBool(oi:False)
if self.Selection.CallEx('InsertFile') ! Insert the file
return True
else
return False
end
The .params property of each oiObject is an instance of the oiParams
class. This allows any number of parameters to be "inserted" before
calling the CallEx() method to call the method. This approach can also be
used to pass parameters to Get and Set calls by specifying memberType
parameter as a value other than oi:DISPATCH_METHOD, which is the default.
Other support values are:
oi:DISPATCH_PROPERTYGET equate(02h) - Get a property
oi:DISPATCH_PROPERTYPUT equate(04h) - Set a property
oi:DISPATCH_PROPERTYPUTREF equate(08h) - Set a property reference
In addition an oiVariant can be passed to the method using the vtRet
parameter to receive the return value from the call.
How To: Moving Mail Betweeen Folders
The code below demonstrates how to move items between folders. This is
based on the actual code in the
MoveItem method provided by
the
oiOutlook class (of course, using
the
MoveItem method is far simpler is this specific case).
Also note that the oiOutlook object now provides a
GetNameSpace method, so the namespace no longer needs to be
fetched manually, as well as methods to retrieve the Folders collection etc.
This specific example is intended purely to demonstrate the use of the
oiObject class.
Folders oiObject ! The Folders collection
InboxFolder oiObject ! The default Inbox (the source folder in this case)
Items oiObject ! A collection of items in the source folder
DestFolder oiObject ! The destination Folder
MailItem oiObject ! Each item that is processed from the source folder
searchString string(200) ! The search string for matching against a Mail field
destFolderName string(80) ! The name of the destination folder
code
! The root item in Outlook is a "NameSpace", which is identified by the string 'MAPI'
! In these examples the namespace is fetched each time a routine is called, however
! It would be more efficient to fetch it once, and then use it as needed, and only
! call the Kill method when done (or allow the object to do this automatically when
! it goes out of scope).
! Get the default MAPI namespace, and then get the default Inbox
ident = 'MAPI'
if Outlook.iApplication.GetChild('GetNamespace', Namespace, ident)
if InboxFolder.Init('GetDefaultFolder', Namespace, oio:FolderInbox)
! Get the Folders collection that is the parent of the default inbox
if Folders.Init('Folders', InboxFolder) and InboxFolder.GetChild('Items', Items)
destFolderName = 'Test'
if Folders.GetChild('Item', DestFolder, destFolderName)
! Find all mail messages with a specific subject line
searchString = '[Subject]="Test Email From Office Inside"'
if not Items.GetChild('Find', MailItem, searchString)
Message('No matching Mail Items were found.', 'No mail to move')
do Cleanup
exit
end
! Move matching mail items to the DestFolder folder
loop
! Found mail item, move it to the Test folde
MailItem.Call('Move', DestFolder)
MailItem.Kill() ! Clean up for the next item
if not Items.GetChild('FindNext', MailItem)
! Loop until there are no more matching items
break
end
end
end
else
Message('The specified destination folder does not exist')
end
end
end
do MailCleanup
! Cleanup: In this case we clean up explicitly, as these routines take a "once off" approach.
! In actual code the object would typically be used as many times and needed, and the Kill
! method only called if an object needs to be reused for another purpose (getting a different item for example)
! or the object is no longer used. If the Kill method is not explicitly called, then it will be called
! when the object goes out of scope (the procedure that it is declared in exits for example).
MailCleanup routine
NameSpace.Kill()
InboxFolder.Kill()
Folders.Kill()
Items.Kill()
DestFolder.Kill()
MailItem.kill()
How To: Send and Receive for all Accounts.
This example demonstrates using the SyncObject objects
to perform a Send and Receive for all accounts.
i long
SyncCol oiObject
SyncObj oiObject
numAccs long
Outlook oiOulook ! Typically populated by the template
code
if Outlook.GetNameSpace()
if Outlook.pNamespace.GetChild('SyncObjects', SyncCol) ! Get the specific appointment object
SyncCol.GetProperty('Count', numAccs)
loop i = 1 to numAccess
if SyncCol.GetChild('SyncObject', SyncObj, i)
SyncCol.CallMethod('Start')
SyncObj.Kill()
else
break
end
end
else
Message('Could not get the SyncObjects collection from Outlook, cannot send and receive')
end
end
SyncCol.Kill()
FAQ (Frequently Asked Questions)
How do I record a macro in Word
At the time of writing this document, Word 2010, using the View menu:
Select the 'Record Macro' from the ribbon bar:
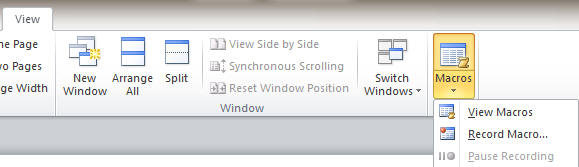
You will then be able to record what you want to do. At the end of doing the
routine you're wanting to do, select the Stop Recording option from the same
menu and then Click View Macros, and then highlight the macro you just recorded
and click edit. This will take you to the Macro editor and display the VBA
script that you can then use to convert to Clarion code (see
Converting a Macro from VBA to Clarion using
OfficeInside for more detail).